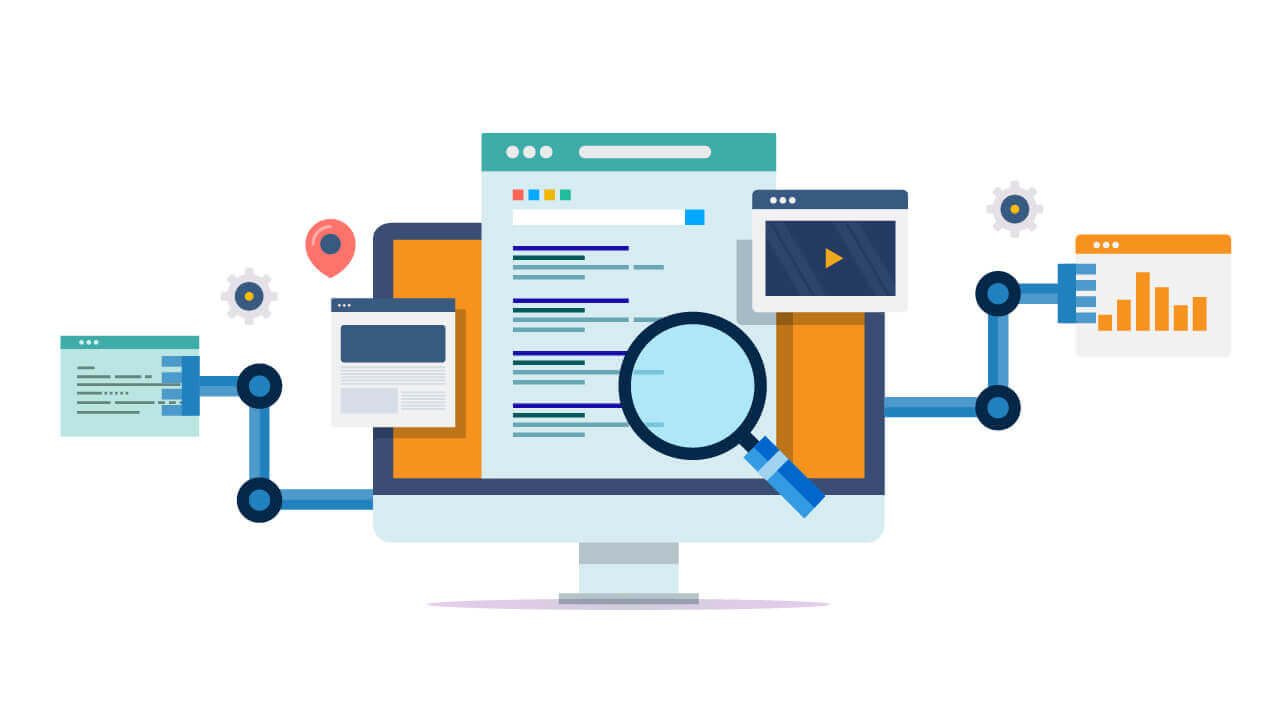
Agile Maturity Through DevOps Testing Excellence
DevOps Testing for Agile Teams is a collaborative and continuous approach to testing that integrates seamlessly with the Agile development process. It emphasizes the importance of automation, continuous integration, and continuous delivery, enabling teams to test software more frequently and efficiently. This approach fosters a culture where development, testing, and operations teams work closely together, breaking down traditional silos. By incorporating testing early and often in the development lifecycle, Agile teams can identify and address issues more quickly, enhance software quality, and accelerate the delivery of value to customers. DevOps Testing is pivotal in supporting a more responsive, adaptive, and quality-focused software development process. To gain deeper insights and assess your team’s agility, consider taking our Team Agility Advanced Assessment at Lean Agile Intelligence.
DevOps Testing and Agile Maturity:
At Lean Agile Intelligence, we recognize DevOps Testing as a cornerstone of Agile success. Our maturity model consists of four stages—Developing, Emerging, Adapting, and Optimizing—designed to guide teams in mastering DevOps Testing. In this post, we’ll explore each stage in depth, offering insights and strategies to help you strengthen your testing practices and achieve higher levels of efficiency and quality.
* * * * * *
"By incorporating testing early and often in the development lifecycle, Agile teams can identify and address issues more quickly, enhance software quality, and accelerate the delivery of value to customers."
* * * * * *
Developing
An agile team “developing” an understanding of the value of DevOps Testing and adopting the foundational techniques should focus on the following improvements:
- The What: Testing occurs in a separate "test" environment with the necessary test data
- The How: By following the five steps below and leveraging the tools mentioned, an Agile team can effectively ensure that testing occurs in a well-maintained, separate test environment with the necessary test data, thereby enhancing the quality and reliability of the software development process.
-
Environment Setup:
- Replicate Production: Create a test environment that closely mirrors the production environment. This includes similar hardware, software, network configurations, and security settings.
- Infrastructure as Code (IaC): Use tools like Terraform, AWS CloudFormation, or Ansible to automate the setup and maintenance of your test environment, ensuring consistency and efficiency.
-
Test Data Management:
- Data Masking and Anonymization: If using production data, ensure it's anonymized to protect sensitive information. Tools like Delphix or Informatica can be used for data masking.
- Synthetic Data Generation: Alternatively, use tools like Faker, GenerateData, or Mockaroo to create realistic, synthetic test data that doesn’t carry privacy concerns.
-
Continuous Integration and Deployment:
-
Automated Testing:
- Test Automation Tools: Implement automated testing tools such as JUnit, Cucumber, or TestNG for functional testing, and JMeter or Gatling for performance testing.
- Continuous Testing: Integrate automated tests into your CI/CD pipeline, so tests are run automatically every time code is deployed to the test environment.
-
Monitoring and Feedback:
- Monitoring Tools: Use monitoring tools like Prometheus, Grafana, or New Relic to monitor the test environment and gather feedback on test executions.
- Feedback Loop: Establish a feedback loop where test results are quickly communicated back to the development team for prompt action.
-
- The How: By following the five steps below and leveraging the tools mentioned, an Agile team can effectively ensure that testing occurs in a well-maintained, separate test environment with the necessary test data, thereby enhancing the quality and reliability of the software development process.
- The What: Automated unit tests exist and are run regularly
-
- The How: To ensure that an Agile team runs automated unit tests regularly, a few practical tips can be integrated into their workflow.
- First, it's essential to integrate the unit testing process into the continuous integration (CI) pipeline. Tools like Jenkins, CircleCI, or Travis CI can be set up to automatically trigger unit tests every time code is committed or merged into the main branch. This ensures that tests are run consistently and automatically, reducing the risk of human error or oversight.
- Another key practice is to make unit testing a part of the definition of done for every user story or task. This means a piece of code is not considered complete until it has corresponding unit tests that pass successfully. This approach embeds testing into the development process, making it a routine part of the team's workflow.
- Fostering a culture that values testing is crucial. Encouraging developers to write tests first, a practice known as Test-Driven Development (TDD), can be very effective. In TDD, developers first write a failing test based on the requirement, then write the code to pass the test, and finally refactor the code while ensuring the test still passes. This not only ensures that testing is an integral part of development but also helps in writing cleaner, more efficient code.
- Regularly reviewing and refactoring tests is also important. Just like code, tests need to be maintained. This involves updating tests to reflect changes in the application, removing obsolete tests, and improving test coverage and efficiency.
- The How: To ensure that an Agile team runs automated unit tests regularly, a few practical tips can be integrated into their workflow.
Emerging
An agile team “emerging” beyond the understanding of the value of DevOps Testing and adopting the foundational techniques should focus on the following improvements:
- The What: Automated unit and integration tests are triggered during the build process
- The How: A key tip to ensure that automated unit and integration tests are triggered during the build process is to integrate your testing framework with a Continuous Integration (CI) tool. For instance, if you're using a CI tool like Jenkins, you can configure your build pipeline to automatically execute unit and integration tests each time a build is initiated.
- Here's how you can achieve this:
- Configure the Build Pipeline: In your CI tool (e.g., Jenkins), set up a build pipeline for your project. Within this pipeline, define a step or job specifically for running tests.
- Script Integration: In the build script (like a pom.xml file for Maven projects, or a build.gradle file for Gradle projects), include commands to run your unit and integration tests. For example, in a Maven project, you might use mvn test to trigger the tests.
- Automate Triggering: Configure the CI tool to trigger this build pipeline automatically upon code commits to your version control system (like Git). This ensures that every change in the codebase initiates a new build, along with the execution of your tests.
Recommended Blog Post From Headspin
Adapting
An agile team “adapting” beyond the understanding of the value of DevOps Testing and adopting the foundational techniques should focus on the following improvements:
- The What: Feature automated acceptance tests are triggered during the build process
- The How: Integrating Behavior-Driven Development (BDD) with tools like Cucumber into your build process for automated acceptance testing involves several practical steps.
- Here's how an Agile team can achieve this:
- Write Feature Files in Gherkin: Start by writing your acceptance criteria as feature files in Gherkin language, which is used by Cucumber. These feature files should describe the desired behavior of the application in a clear, concise, and readable format for both technical and non-technical team members.
- Implement Step Definitions: For each step in your Gherkin feature files, write corresponding step definitions in your programming language. These step definitions are the actual test code that Cucumber will execute.
- Integrate Cucumber with Your Build Tool: Configure your build tool (like Maven, Gradle, or Jenkins) to run Cucumber tests. For instance, in a Maven project, you can configure the pom.xml to include the Cucumber plugin and specify the path to your feature files and step definitions.
- Automate Test Execution in CI Pipeline: In your Continuous Integration (CI) pipeline, set up a job or stage to execute the Cucumber tests. This can be done by adding a script in your CI configuration that runs the Cucumber tests as part of the build process. For example, a command like mvn test in a Maven project or gradle test in a Gradle project.
- Maintain and Refactor Tests Regularly: As your application evolves, regularly update and refactor your feature files and step definitions to keep them relevant and maintainable. This ensures your acceptance tests accurately reflect the current state of your application.
- Below is an example of a Behavior-Driven Development (BDD) test for a user story about adding items to a shopping cart. The test is written in Gherkin language, which Cucumber uses for BDD tests.
- User Story: Add Items to Shopping Cart
Scenario: Customer adds an item to the cart
Given I am on the product page for "Blue Widget"
When I click on "Add to Cart"
Then "1x Blue Widget" should be added to the cart
And the cart total should update accordinglyScenario: Customer adds multiple quantities of an item to the cart
Given I am on the product page for "Red Widget"
When I select "3" from the quantity dropdown
And I click on "Add to Cart"
Then "3x Red Widget" should be added to the cart
And the cart total should update to reflect the quantityScenario: Customer adds an item to the cart from the product list
Given I am on the product list page
When I click on "Add to Cart" for "Green Widget"
Then "1x Green Widget" should be added to the cart
And the cart total should update accordinglyScenario: Customer tries to add an out-of-stock item to the cart
Given I am on the product page for "Yellow Widget"
And "Yellow Widget" is out of stock
When I attempt to click on "Add to Cart"
Then the "Add to Cart" button should be disabled
And a message "Item Out of Stock" should be displayed
- User Story: Add Items to Shopping Cart
- Here's how an Agile team can achieve this:
- The How: Integrating Behavior-Driven Development (BDD) with tools like Cucumber into your build process for automated acceptance testing involves several practical steps.
- The What: Automated test suites enforce quality gates
- The How: A quality gate is a checkpoint in the software development process where certain criteria must be met before the project can proceed to the next phase. An example of a quality gate is the "Code Review and Static Analysis Gate" in the Continuous Integration (CI) pipeline. In this quality gate, before any new code is merged into the main branch, it must undergo a thorough code review process and pass static code analysis.
- The criteria for passing this gate could include:
- Code Review Approval: The new code changes must be reviewed and approved by at least one other developer, preferably someone with expertise in the area of the code. This review ensures adherence to coding standards, checks for logical errors, and verifies that the new code integrates well with the existing codebase.
- Static Code Analysis: The code is automatically analyzed using tools like SonarQube, ESLint, or FindBugs. These tools scan the code for potential security vulnerabilities, code smells, and programming errors. The code must pass the analysis with no critical issues reported.
- Coding Standards Compliance: The code must adhere to predefined coding standards and guidelines. This could include naming conventions, formatting rules, and best practices for code structure.
- Automated Tests Pass: Any existing automated unit or integration tests must pass with the new code changes. This ensures that the new code does not break any existing functionality. When these criteria are met, the code can only be merged into the main branch. This quality gate helps maintain code quality, improving code maintainability and reducing the likelihood of defects in the production environment.
Optimizing
An agile team “optimizing” beyond the understanding of the value of DevOps Testing adopting the foundational techniques should focus on the following improvements:
- The What: Automated performance testing
- The How: Automated performance testing is a crucial aspect of ensuring the scalability and reliability of applications, especially in continuous integration/continuous deployment (CI/CD) pipelines. Several tools are widely used for this purpose, each with its own strengths and capabilities.
- One popular tool for performance testing is Apache JMeter. It's an open-source application designed for load testing and measuring performance. JMeter is highly versatile, allowing tests against different server types (such as HTTP, HTTPS, SOAP, and JDBC). Another powerful tool is Gatling, known for its high performance and detailed reporting capabilities. Gatling uses a DSL for test scripting, making it more accessible for developers unfamiliar with traditional performance testing tools. For teams working in a .NET environment, tools like LoadComplete or NeoLoad offer comprehensive solutions for creating and executing performance tests and analyzing results.
- To effectively integrate performance testing into a CI/CD pipeline, consider the following tips:
- Automate Test Triggering: Configure your CI server (like Jenkins, CircleCI, or Travis CI) to trigger performance tests automatically at critical pipeline stages. Commonly, performance tests are run after successful deployment to a staging environment, where the application is close to the production setup. This ensures that performance issues are caught before the application is deployed to production.
- Manage Test Data and Environments: Ensure the environment where tests are run is as close to production as possible. This includes the server setup, database, and network configuration. Additionally, use realistic test data to simulate actual usage scenarios accurately.
- Monitor and Analyze Results: Integrate your performance testing tools with monitoring solutions to gather detailed insights. Tools like Grafana or Prometheus can visualize test results and track performance over time. Set up alerts for performance degradation to quickly identify and address issues.
- Iterate and Evolve Tests: Regularly review and update your performance tests to reflect changes in the application and emerging usage patterns. Performance testing should be an ongoing process, adapting as the application evolves and grows.
- The What: Automated security testing
- The How: Automated security testing is an essential component of a robust CI/CD pipeline, ensuring that applications are functional and secure from potential threats. Several tools are explicitly designed for this purpose, each catering to different aspects of security testing.
- For static application security testing (SAST), tools like SonarQube, Checkmarx, and Fortify comprehensively analyze source code for potential security vulnerabilities. These tools can scan code repositories and integrate seamlessly with CI tools like Jenkins or Travis CI, for dynamic application security testing (DAST), which tests running applications. Tools like OWASP ZAP and Burp Suite are popular. They can automatically crawl through your web applications and identify issues like SQL injection, cross-site scripting, and other common vulnerabilities.
- When implementing automated security testing in a CI/CD pipeline, consider the following tips:
- Integrate Early in the Development Process: Security testing should be integrated as early as possible in the development lifecycle. This means configuring your CI tools to run SAST tools with every code commit or at least daily. By identifying vulnerabilities early, you can address them before they become more complex and costly.
- Incorporate DAST in Later Stages: DAST should be performed on deployed applications in a staging environment that closely mirrors production. This can be automated to occur after deployment in the CI/CD pipeline, ensuring that the running application is tested for vulnerabilities before it goes live.
- Manage False Positives and Prioritize Fixes: Automated security tools can sometimes generate false positives. It’s essential to review and triage the results, prioritizing fixes based on the severity and potential impact of the vulnerabilities. Integrating these tools with issue-tracking systems can help manage and track these vulnerabilities effectively.
- Keep Security Tools Updated: Security threats are constantly evolving, so it's crucial to keep your security testing tools updated with the latest threat databases and testing algorithms. Regular updates will help identify new and emerging security vulnerabilities.
- Educate and Collaborate: Foster a culture of security awareness within your development team. Developers should be trained to understand common security threats and best practices. Collaboration between security and development teams is key to effectively addressing and mitigating security issues.
* * * * * *
"Automated security tools can sometimes generate false positives. It’s essential to review and triage the results, prioritizing fixes based on the severity and potential impact of the vulnerabilities."
* * * * * *
Conclusion:
Adopting DevOps Testing in Agile environments transforms the way teams collaborate and approach software quality. By progressing through the stages of Developing, Emerging, Adapting, and Optimizing, teams can fully integrate testing into their CI/CD pipelines and build a culture of continuous improvement. This approach not only enhances software quality but also accelerates delivery, resulting in better customer satisfaction and increased responsiveness to change. To evaluate your team’s current practices and uncover strategies for improvement, take our Team Agility Advanced Assessment at Lean Agile Intelligence and empower your team to reach new heights in agility.